My Skills
A comprehensive set of technical skills I've developed through years of experience and continuous learning.
Always Learning
The tech world evolves rapidly, and I'm committed to staying at the forefront. Currently exploring: AI integration, Web3 technologies, and advanced animation techniques.
My Services
Specialized services tailored to meet your development needs with modern technologies and best practices.
Client Testimonials
Don't just take my word for it. Here's what my clients have to say about working with me.

Sarah Johnson
Product Manager at TechCorp
Working with Naim was an absolute pleasure. His technical expertise and attention to detail resulted in a product that exceeded our expectations. He's not just a developer, but a problem solver who truly understands business needs.
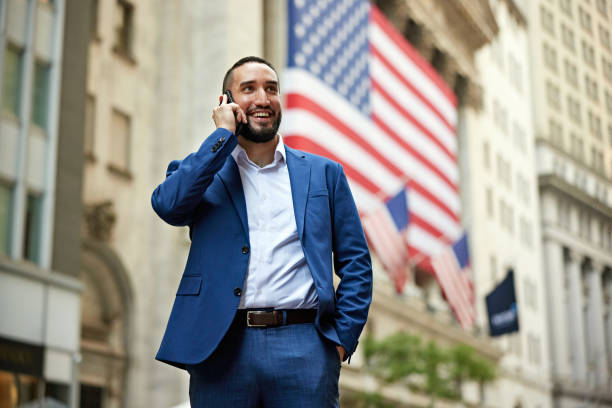
Michael Chen
Startup Founder at InnovateLabs
Naim helped transform our idea into a fully functional platform in record time. His ability to suggest improvements and implement complex features made all the difference. I highly recommend his services to anyone looking for quality development work.
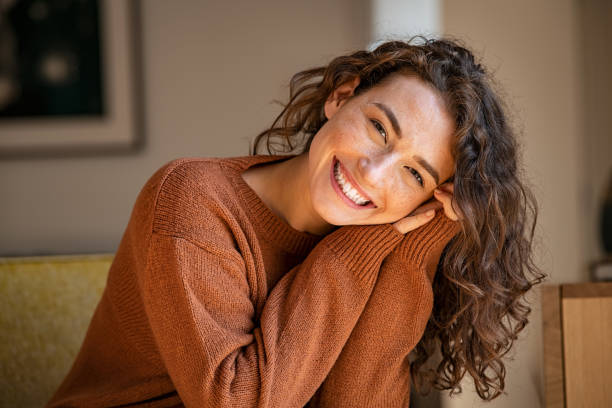
Emily Rodriguez
Marketing Director at CreativeEdge
Our website redesign project was in capable hands with Naim. He understood our brand vision perfectly and delivered a responsive, modern site that has significantly improved our conversion rates. A true professional who delivers on time and on budget.